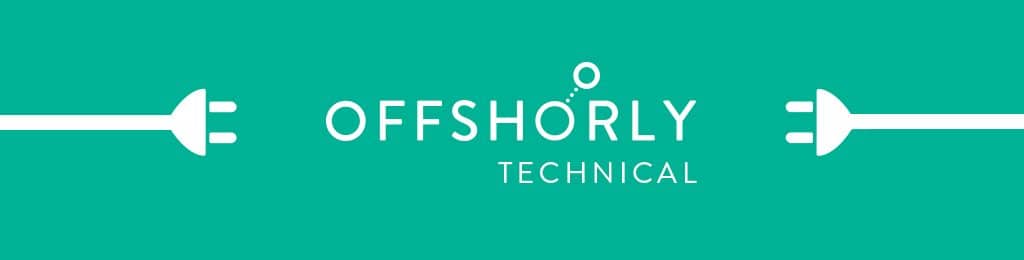
Lodash is a reference library made with JavaScript. In addition, it’s the most famous successor of underscore.js.
It is used for simplifying the handling and edition of objects, arrangements, etc. since this provides many useful methods to do so. At the same time, simplifying this work meaning our code is more legible and easy to follow for third parties.
Here are some examples of comparison between traditional way of coding vs using Lodash library:
1. Return the value of a key of the first item of an array that is the value of another key:
// Get the name of the first brother of each person
var persons = [{
“name”: “Jose Luis”,
“brothers”: [{“name”:”David”}, {“name”: “Sonia”}]
}, {
“name”: “Alfonso Manuel”,
“brothers”: [{“name”:”Gustavo”}, {“name”: “Juan”}]
}];
// Array’s map method.
var names = persons.map(function(item){
return item.brothers[0].name;
});
// [ ‘David’, ‘Gustavo’ ]
// Lodash
var namesBis = _.map(persons, ‘brothers[0].name’);
// [ ‘David’, ‘Gustavo’ ]
2. Get a random number between two numbers:
// Get a random number between 15 and 20.
// Native Function
function getRandomNumber(min, max){
return Math.floor(Math.random() * (max – min + 1)) + min;
}
var random = getRandomNumber(15, 20);
// ej: 16
// Lodash
var randomTwo = _.random(15, 20);
// ej: 19
In conclusion, Lodash can be a very helpful library for developers as it has a lot of utility methods/functions that are common to programming, simplified and easy to use.
For more information, you can visit their documentation here: https://lodash.com/